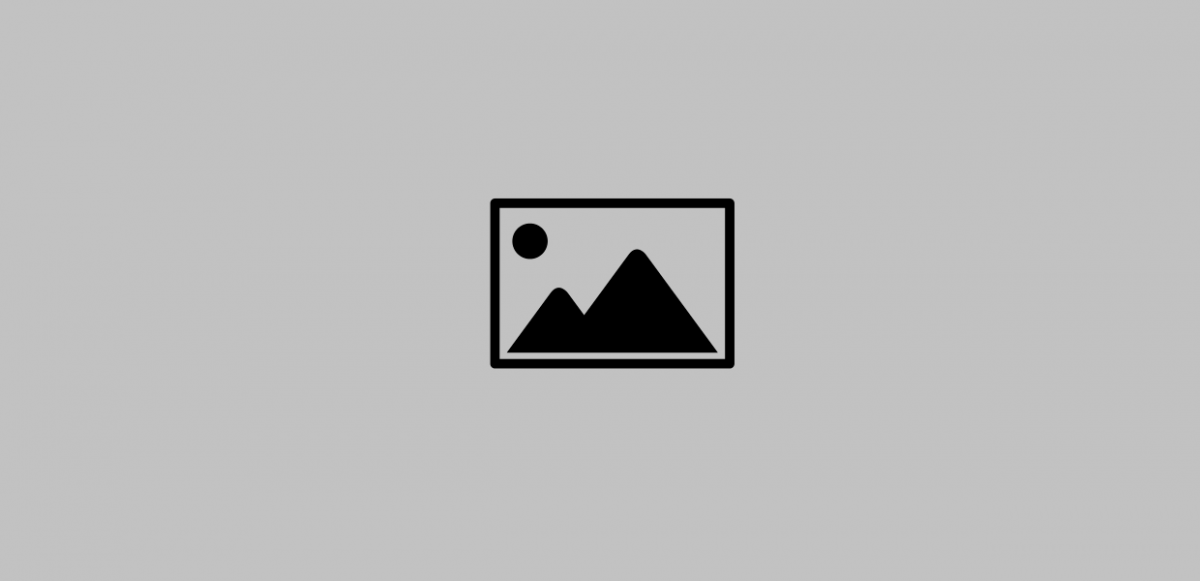
Writing Out LINQ to XML Documents to CSV Text Files
This may sound stupid, but I wanted to store some text parsing I was doing for some VDP projects in LINQ to XML XDocuments.
So, I created the following:
XDocument recs = new XDocument();
XElement records1 = new XElement("Records");
XElement record1 = new XElement("Record");
record1.Add(new XElement("id", "1"),
new XElement("Name","Test"),
new XElement("Address","123 Anywhere")
);
records1.Add(record1);
recs.Add(records1);
which should create an xml document looking like this:
<Records>
<Record>
<id>1</id>
<Name>Test</Name>
<Address>123 Anywhere</Address>
</Record>
</Records>
So, I want to write this out to a CSV file. But I want to assume that my XML Nodes might change (but at least stay consistent throughout the XML Document) by datasource and I want to systematically write out the names of the nodes and then loop through and write out the values.
So the hard part:
var list2 = from el2 in recs.Elements("Record")
select el2;
//Build Header Line
StringBuilder csvlist = new StringBuilder();
var headers = (list2.First().Nodes().ToList());
int cc = 1;
foreach (var node in headers)
{
csvlist.Append((node as XElement).Name);
if (cc != headers.Count)
{
csvlist.Append(",");
}
cc++;
}
csvlist.AppendLine();
foreach (var t in list2)
{
for (int i = 0; i < t.Nodes().Count(); i++)
{
csvlist.Append((t.Nodes().ToList()[i] as XElement).Value);
if (i != (t.Nodes().Count() - 1))
{
csvlist.Append(",");
}
}
csvlist.AppendLine();
}
So at the end of all this, csvlist contains the headers and data as comma separated.
There are lots we could add or fix in this, but it allows us great flexibility in outputting xml data as delimited text.
DISCLAIMER: I am not a professional coder, I do this as a passion. The above code has no warranty or ability to actually do what I say it does. Use at your own risk, fix, add or change if you are willing.