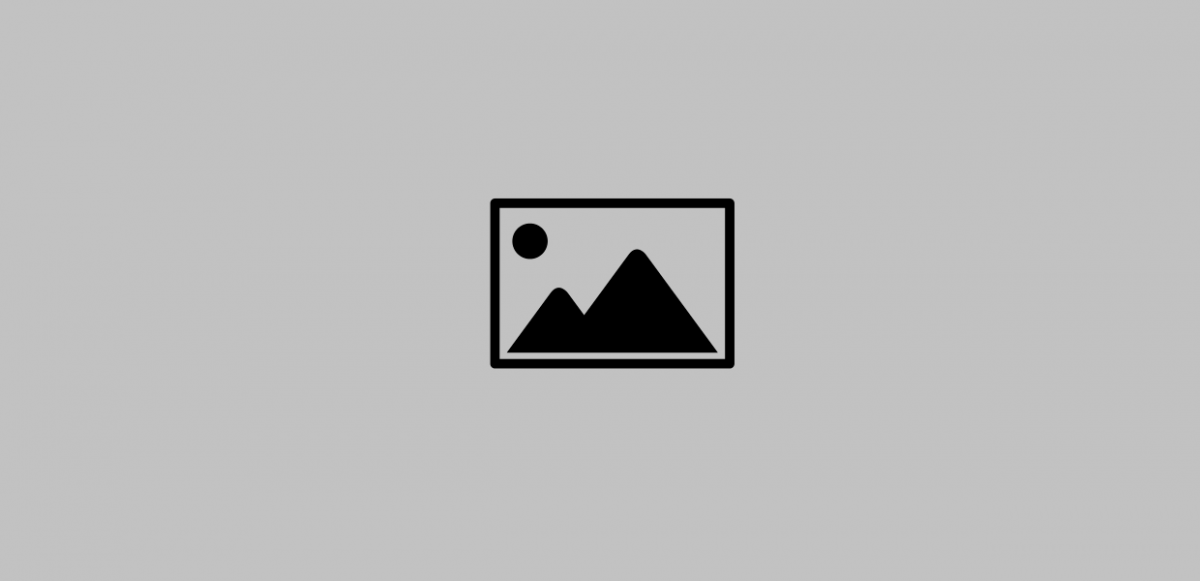
WPF Dynamically Added Controls and Getting Values
Today’s challenge was fun.
I had added a bunch of textboxes and labels to a Grid with 2 StackPanel in my code behind.
StackPanel sp1 = new StackPanel();
StackPanel sp2 = new StackPanel();
Grid g = new Grid();
ColumnDefinition colDef1 = new ColumnDefinition {Width = new GridLength(50)};
ColumnDefinition colDef2 = new ColumnDefinition();
g.ColumnDefinitions.Add(colDef1);
g.ColumnDefinitions.Add(colDef2);
gb.Content = g;
Grid.SetColumn(sp1,0);
g.Children.Add(sp1);
Grid.SetColumn(sp2, 1);
g.Children.Add(sp2);
for (int i = 1, i < = 4, i++)
{
Label lbl = new Label {Content = "Name", Margin = new Thickness(0)};
Grid.SetColumn(lbl, 0);
sp1.Children.Add(lbl);
TextBox fieldname = new TextBox {Text = "", Name = "fieldname" + i, Margin = new Thickness(0,5,0,0)};
Grid.SetColumn(fieldname, 1);
sp2.Children.Add(fieldname);
}
So I then wanted to get the results of what someone types into these TextBoxs.
But wait, I can’t just access them like this:
string t = fieldname1.Text;
Why? Only Microsoft knows, but apparently, dynamically added controls can’t be accessed this way since they don’t exist in the XAML code.
So, how does one access them then? Well, that’s where this cool LogicalTreeHelper function to find the control by name we use the FindLogicalNode option of the LogicalTreeHelper function
So to loop through the number of fields you added you can do the following:
List test = new List;
for (int i = 1; i < = 4; i++)
{
test.Add((LogicalTreeHelper.FindLogicalNode(Fields1, "fieldname" + i) as TextBox).Text);
}
So there you have it, a simple way to access dynamically added controls using LogicalTreeHelper and FindLogicalNode just remember to name your controls you add.